Important Note: The intended audience for this article are software developers, with an intermediate level (2+ years) understanding of Internet and programming concepts.
Previously, we introduced the concept of Software License Managers (SLM); and the important role they play, both in increasing product sales and protecting intellectual property.
In this article, we’re going to discuss how a typical software product communicates with an SLM; specifically the Software License Manager Plugin for WordPress.  All further references to the term SLM will refer to this specific plugin.
You’ve Got a Secret
You can’t allow just anyone to communicate with the SLM.  When the SLM is first installed, it creates 2 secret keys:
- The “License Creation” secret key, used to seed the license key generator.
- The “License Verification” secret key, used to authenticate license activation/deactivation messages.
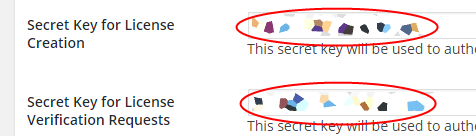
The License Verification key is the only way for the SLM “to know” that a message is really coming from a product, that is authorized to communicate with the SLM. Â It also means that if you ever reinstall the SLM on another server (using the same domain name), you must use the same key; otherwise previously licensed products will be unable to properly communicate with the SLM. Â So, WRITE IT DOWN.
Only you, the SLM and any software products that use that specific SLM should know the License Verification key.  For high value products, written in human readable code (PHP or Javascript), you might consider the use of a code obfuscation tool to ensure the security of the License Verification key.
As briefly noted, once you begin distributing your product; not only must you use the same License Verification key, but also the same Internet domain/URL for the SLM.  Once you begin distributing your licensed products; the License Verification key and the Internet URL for the SLM must never change.  Keeping the URL used by the SLM a secret, is not critical; but it is best not to publicize what the URL is, in order to minimize Denial of Service attacks.
Talk to Me
Products communicate with the SLM using the HTTP Get or POST method.  If the product is say, a WordPress plugin; you might use the wp_remote_get() function.  Or you could use the more secure HTTP POST method and communicate with the SLM using the PHP curl_exec() function.  If your product is an Android application you’d use the HttpURLConnection class; or  the NSURLConnection class for iOS.
All responses from the SLM are in the form of a JSON (JavaScript Object Notation) message:
{ "result" : string , "message" : string }
Let’s Play Computer
The best way to understand the messaging process, is to manually practice passing messages to the SLM using your Internet browser; allowing you to emulate the HTTP Get method.  The specific values for the required parameters, used in these examples will differ from your own.
Prerequisites
Manually create a license key on the SLM, using the “Add/Edit Licenses” menu.  You’ll also need to know the URL of the SLM, and its License Verification secret key.
- slm_url = http://example.com/wp
This is the URL of your SLM. - secret_key =Â 55622f04c0ce17.41911005
This is the License Verification secret key. - license_key =Â 556bbc693638d
- This is a license key that has been issued to a customer.
- Typically, the customer would be prompted, via a product settings form, for this information. Â The information is needed to both activate and deactivate the product license.
- registered_domain = “”
- If you were going to lock a license to a specific domain, as in the case of a WordPress plugin product, this would be the URL of the customer’s WordPress installation.
- In the case of an Android or iOS application, this parameter should be an empty string.
- DO NOT omit this parameter, as the default NULL string IS NOT EQUAL to an “empty string.”
Example of Retrieve License Details and Check it
The following HTTP Get will retrieve the details of a key so you can check it:
http://example.com/wp/?secret_key=55622f04c0ce17.41911005&slm_action=slm_check&license_key=556bbc693638d
{"result":"success","message":"License key details retrieved.","status":"active"}
It will output the full details of the license key.
License Activation Example
The following HTTP Get will activate a license key:
http://example.com/wp/?secret_key=55622f04c0ce17.41911005&slm_action=slm_activate&license_key=556bbc693638d®istered_domain=""
{"result":"success","message":"License key activated"}
And if the license is activated too many times, an error occurs:
{"result":"error","message":"Reached maximum allowable domains"}
License Deactivation Example
The following HTTP Get will deactivate a license key:
http://example.com/wp/?secret_key=55622f04c0ce17.41911005&slm_action=slm_deactivate&license_key=556bbc693638d®istered_domain=""
{"result":"success","message":"The license key has been deactivated for this domain"}
And if the license is deactivated too many times, a warning will be returned:
{"result":"error","message":"The license key on this domain is already inactive"}
Now that you’ve mastered the basics of manually communicating with the SLM, using a browser to emulate messages sent/received with the HTTP Get method; you can feel confident using programming functions and classes, to integrate SLM communications into your software products.
Thanks admin, didn’t see that. works a treat now.
@Pete, Have you done step 4 from the following documentation? That email shortcode will add the license key in the purchase notification.
https://www.tipsandtricks-hq.com/ecommerce/integrate-wp-estore-with-software-license-manager-plugin-3731
A license key is generated automatically from a purchase through the WP eStore plugin but the “License Key” is not being sent in the purchase confirmation email message from the WP eStore. How should the customer get the license key sent?