This documentation is only for developers. If you are not a developer with good PHP coding skills then you have no need to read this.
This documentation explains how you can capture a lead from your own script using the API of the affiliate plugin. If you want to use this API then you need to enable it from the settings menu of the affiliate plugin first. The following is a screenshot of this section in the settings menu:
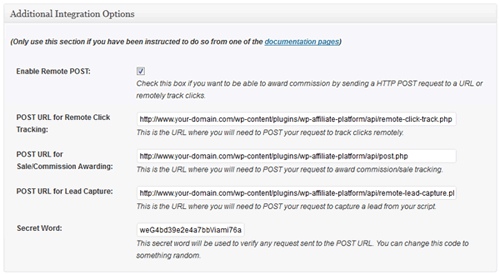
Additional Integration Options Screenshot
Once you enable it, you can start to send request to the specified URL from your script to capture lead remotely.
You need a minimum of 3 pieces of information to capture a lead using a HTTP request. These are:
- Secret word (the secret word that you have specified in the settings)
- Referrer ID (the affiliate ID of the referrer who sent this visitor your way)
- Email address of the lead/customer
Optionally, you can pass in the following additional data too:
- A reference value (this can help you identify the source if you are capturing lead from multiple places
- IP address of the lead
1. Capturing Lead via HTTP GET request
To capture a lead via HTTP GET use the following format:
http://www.example.com/wp-content/plugins/wp-affiliate-platform/api/remote-lead-capture.php?secret=XX&ap_id=YY&buyer_email=ZZ
Replace the “example.com”, “XX”, “YY”, “ZZ” with the appropriate value.
PHP Code Example
The following is an example of how to construct this link using PHP:
$secret = "4bd39e2e4a7bb"; $referrer = "aff21"; $email = "[email protected]"; $prepared_data = "?secret=".$secret."&ap_id=".$referrer."&buyer_email=".$email; $get_url = "http://www.example.com/wp-content/plugins/wp-affiliate-platform/api/remote-lead-capture.php".$prepared_data; // Execute this GET Request file_get_contents($get_url);
2. Capture Lead via HTTP POST request
To capture lead via HTTP POST use the following format:
<form method="post" action="http://www.example.com/wp-content/plugins/wp-affiliate-platform/api/remote-lead-capture.php"> <input type="hidden" name="secret" value="XX"> <input type="hidden" name="ap_id" value="YY"> <input type="hidden" name="buyer_email" value="ZZ"> <input type=submit value="Submit Post"> </form>
Replace the “example.com”, “XX”, “YY”, “ZZ” with the appropriate value.
PHP Code Example
If you are integrating the affiliate platform plugin with a script written in PHP then you can add the following example code to capture the lead when your script executes:
How to Programmatically Retrieve the Affiliate ID on a Page
If you are writing a script then you most likely want to code it up so the affiliate ID can be retrieved programmatically. The affiliate ID is stored in the cookie so just retrieve the value form the cookie. For example the following code will give you the affiliate ID if used in PHP:
$affiliate_id = $_COOKIE['ap_id'];
Now, you can use the “$affiliate_id” variable in your script.
Leave a Reply