This documentation is only for developers. If you are not a developer with good PHP coding skills then you have no need to read this.
It is very hard to troubleshoot another coder’s code. So if you use this documentation and do a custom integration yourself then please note that we cannot offer you any support in terms of what is wrong with your code. You will need to hire us to take a look at your code and correct any mistakes you made.
You can also retrieve referrer details using shortcode offered by this plugin (this doesn’t require you to use the API).
This API allows you to retrieve a particular info from your affiliate’s profile via a HTTP GET or POST request. If you want to use this API then you need to enable it from the settings menu. The following is a screenshot of this section in the “Integration Related” settings menu:
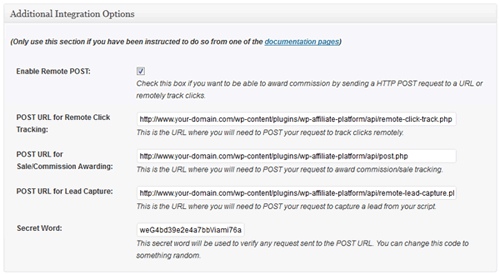
Additional Integration Options Screenshot
Once you enable it, you can start to send request to a URL to retrieve affiliate’s info programmatically.
You need a minimum of 3 pieces of information to do this via a HTTP request. These are:
- Secret word (the secret word that you have specified in the settings)
- Referrer ID (the affiliate ID)
- Info (the value of the field you want to retrieve)
Option 1. HTTP GET request
To do this via HTTP GET use the following format:
http://www.example.com/wp-content/plugins/wp-affiliate-platform/api/get_user_data.php?secret=XX&ap_id=YY&info=ZZ
Replace the “example.com”, “XX”, “YY”, “ZZ” with the appropriate value.
PHP Code Example
The following is an example of how to construct this link using PHP. The following will retrieve the “email” field value of the affiliate with ID “tips21”
$secret = "4bd39e2e4a7bb"; $referrer = "tips21"; $info = "email"; $prepared_data = "?secret=".$secret."&ap_id=".$referrer."&info=".$info; $get_url = "http://www.example.com/wp-content/plugins/wp-affiliate-platform/api/get_user_data.php".$prepared_data; // Execute this GET Request file_get_contents($get_url);
Option 2. HTTP POST request
To use HTTP POST use the following format:
<form method="post" action="http://www.example.com/wp-content/plugins/wp-affiliate-platform/api/get_user_data.php"> <input type="hidden" name="secret" value="XX"> <input type="hidden" name="ap_id" value="YY"> <input type="hidden" name="info" value="ZZ"> <input type=submit value="Submit Post"> </form>
Replace the “example.com”, “XX”, “YY”, “ZZ” with the appropriate value.
PHP Code Example
The following PHP example code will retrieve the “email” address field value of the affiliate with ID “tips21”
$affiliate_id = "tips21"; // The affiliate ID of the user in question $info = "email"; //What info to retrieve. In this case, the email address. // The Post URL (replace "example.com" with your actual domain-name) $postURL = "http://www.example.com/wp-content/plugins/wp-affiliate-platform/api/get_user_data.php"; // The Secret key (Get this value from the settings menu of this plugin) $secret_key = "4bc67180656782.45045137"; // Prepare the data $data = array (); $data['secret'] = $secret_key; $data['ap_id'] = $affiliate_id; $data['info'] = $info; // send data to post URL to award the commission $ch = curl_init ($postURL); curl_setopt ($ch, CURLOPT_POST, true); curl_setopt ($ch, CURLOPT_POSTFIELDS, $data); curl_setopt ($ch, CURLOPT_RETURNTRANSFER, true); $returnValue = curl_exec ($ch); curl_close($ch);
If the affiliate ID you specified in the request is invalid (doesn’t exist), then it will show an error. Otherwise, it will show the value of the field.
Leave a Reply