This documentation is only for developers. If you are not a developer with good PHP coding skills then you have no need to read this.
It is very hard to troubleshoot another coder’s code. So if you use this documentation and do a custom integration yourself then please note that we cannot offer you any support in terms of what is wrong with your code. You will need to hire us to take a look at your code and correct any mistakes you made.
Depending on your situation you may need to be able to award commission via a HTTP GET or POST request. WP Affiliate Platform has an API for awarding commission using a standard HTTP GET or POST request. If you want to use this API then you need to enable it from the settings menu. The following is a screenshot of this section in the settings menu:
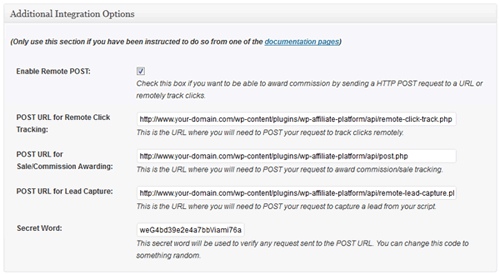
Additional Integration Options Screenshot
Once you enable it, you can start to send request to the specified URL to award commission remotely.
You need a minimum of 3 pieces of information to award commission via a HTTP request. These are:
- Secret word (the secret word that you have specified in the settings)
- Referrer ID (the affiliate ID)
- Sale amount (commission will be calculated based on this amount)
Optionally, you can pass in the following additional data too:
- Transaction ID (txn_id)
- Item ID (item_id)
- Buyer email address (buyer_email)
1. Awarding Commission via HTTP GET request
To award commission via HTTP GET use the following format:
http://www.example.com/wp-content/plugins/wp-affiliate-platform/api/post.php?secret=XX&ap_id=YY&sale_amt=ZZ
Replace the “example.com”, “XX”, “YY”, “ZZ” with the appropriate value.
PHP Code Example
The following is an example of how to construct this link using PHP:
$secret = "4bd39e2e4a7bb"; $referrer = "amin21"; $sale_amt = "29.95"; $prepared_data = "?secret=".$secret."&ap_id=".$referrer."&sale_amt=".$sale_amt; $get_url = "http://www.example.com/wp-content/plugins/wp-affiliate-platform/api/post.php".$prepared_data; // Execute this GET Request file_get_contents($get_url);
2. Awarding Commission via HTTP POST request
To award commission via HTTP POST use the following format:
<form method="post" action="http://www.example.com/wp-content/plugins/wp-affiliate-platform/api/post.php"> <input type="hidden" name="secret" value="XX"> <input type="hidden" name="ap_id" value="YY"> <input type="hidden" name="sale_amt" value="ZZ"> <input type=submit value="Submit Post"> </form>
Replace the “example.com”, “XX”, “YY”, “ZZ” with the appropriate value.
PHP Code Example
If you are integrating the affiliate platform plugin with a Payment Gateway or a Shopping Cart in PHP then you can add the following example code to your post payment processing script or the “Thank You” page script to award commission after a payment:
How to Programmatically Retrieve the Affiliate ID on a Page
If you are writing a script then you most likely want to code it up so the affiliate ID can be retrieved programmatically. The affiliate ID is stored in the cookie so just retrieve the value form the cookie. For example the following code will give you the affiliate ID if used in PHP:
$affiliate_id = $_COOKIE['ap_id'];
Now, you can use the “$affiliate_id” variable.
Calculating Commission in Your Script
Normally, if you send the total sale amount value to the API, it will calculate the commission based on your settings and award it to the affiliate. However, you can choose to calculate the total commission in your script then send that amount to the API. The commission amount you send to the API will be given directly to the affiliate (no additional calculation will be performed).
You can pass the total commission amount value using the “commission_amt” parameter in your API request. Below is an example:
<form method="post" action="http://www.example.com/wp-content/plugins/wp-affiliate-platform/api/post.php"> <input type="hidden" name="secret" value="XX"> <input type="hidden" name="ap_id" value="YY"> <input type="hidden" name="sale_amt" value="ZZ"> <input type="hidden" name="commission_amt" value="AA"> <input type=submit value="Submit Post"> </form>
Replace the “example.com”, “XX”, “YY”, “ZZ”, “AA” with the appropriate value.
You can use the following:
$data['buyer_email'] = "[email protected]";
I should have asked, same as above but what’s the $data code for the buyers email address? Thanks!
You can add them to the $data array like the following
$data['txn_id'] = "UniqueTransactionID";
$data['item_id'] = "Item-ID";
You say you can pass Transaction ID and Item ID – can you give a php example like above on how to do that? Thanks!
The sale_amount is not the commission amount. The sale_amount is how much the product costs. The affiliate plugin will use this amount to calculate how much commission it needs to give to the affiliate in question.
So essentially you get the value of the “sale_amount” from your ecommerce plugin. For example if you made a payment via PayPal then the sale_amount value will be stored in the “mc_gross” field. You just pass in that value and the plugin will do the commission calculation based on the settings value you have specified in that particular affiliate’s profile. For exmaple if that affilaite is suppose to get 30% commission then it will give 30% of the sale_amount as a commission to that affiliate. Does that make sense?
For the sale_amt variable. Can it be a percentage or must it be a fixed value?
If it has to be fixed value, wouldn’t I have to update this variable whenever I change my product price?
If I assign a different commission percentage for a particular affiliate. I would have to create another order page just for these special affiliates.
You rule! Thanks for great plugin and super-fast service.
@Juuth, I have added a new section in this page called “How to Programmatically Retrieve the Affiliate ID on a Page”. This section should answer your question.
Hi Ivy,
I’m very new to this. If i want to use this, what exactly should i do? I guess the secret word (XX) is always the same, as specified in the settings menu. And the sales amount (ZZ) can be taken from the subscription form at hand. But what about the affiliate id (YY)?
Thanks for helping out.
Thanks again!!
I didn’t add an API for lead capturing via HTTP POST or GET because most people use a WordPress contact form plugin to capture a lead and there is an alternative way to do this. This post explains how:
http://www.tipsandtricks-hq.com/forum/topic/capturing-lead-when-used-with-a-wordpress-contact-form-plugin
Ya. I gotcha. Maybe I am just overlooking something but when I was looking at the post.php file it seemed as though you can only add a “sale” not a “lead”. I have sales registering. Lead info is not showing which I understand since my post or get doesn’t include the lead data. And I didn’t notice a process within the post.php for capturing the lead info.
You don’t have to use Contact 7 or any other plugin to use this method of commission/lead capturing.
Remember HTTP GET or POST is standard HTTP protocol so you can use it from any application written in any language.
If we are not using Contact 7 but have a different custom lead form… is there another POST or GET we can throw at some php script to register the lead? I am able to get the “sale” tracked using $.get(), but was wondering if I could throw my lead form data somewhere so it shows up in “WP Affiliate -> Manage Leads”.