The auto downgrade membership account addon lets you automatically downgrade any of your expired or inactive premium member’s account to a free level. This lets your premium members who cancelled the payment to still be able to log into your site and browse content for a Dummy/Free membership level.
Create a Registration Form for a Particular Membership Level
WP eMember uses ONE registration form for all kinds of membership registration. This way you don’t have to create multiple pages with different registration forms and confuse your customers. Full details of how eMember does this is explained here.
Occasionally, you may need to create a registration form for a particular membership level for a special reason. For example, give backdoor entrance to your paid membership site to some special people (this way they won’t have to pay)
Create a Registration Form for a Specific Level
Create a new WordPress page. Then use the following shortcode on this page. It will place a registration form for a particular membership level:
[wp_eMember_registration_form_for level=1]
1 is the membership level ID in this example. Change it to the actual membership level ID value for your system.
Now, when a user registers using this form, he will be signing up for that particular membership level that you specified in the shortcode.
You can also hide this special registration page from the standard WordPress navigation menu.
WP eMember Miscellaneous Tweaks
If you are a developer and want to customize the code or if you want to add conditions around your content then the following code examples should be helpful.
Find out if a member is logged in or not
You can use the following function call to determine if a member is logged in or not. The following function call will return true if a member is logged in (otherwise it will return false)
wp_emember_is_member_logged_in()
You can utilize the above function in the following way to ad code in your theme’s template file to add conditional block (for example: show a special message to your logged in users):
<?php if(wp_emember_is_member_logged_in()) { ?> //Place your special message for members here <?php } ?>
The following example can be useful if you want to show ad to non-members of the site:
<?php if(!wp_emember_is_member_logged_in()) { ?> //Place your ad code here <?php } ?>
The following example can be useful if you want to show a message to a member who belongs to membership level 1:
<?php if(wp_emember_is_member_logged_in('1')) { ?> //Place your special message for members from membership level with ID 1 <?php } ?>
The following PHP code example shows how you can programmatically retrieve the member ID of a member and add condition:
<?php $emember_auth = Emember_Auth::getInstance(); $user_id = $emember_auth->getUserInfo('member_id'); if (!empty($user_id)) { //pleace code that only members can see } ?>
Hiding Post Title From Non Members
We will use the “wp_emember_is_member_logged_in” function (explained above) to modify the “Twenty Eleven” theme and make the post title only visible to users who are logged in.
This is an example for when a post is displayed on a single page – ie, I edited the content-single.php file:
The original line of code was as follows:
<h1 class="entry-title"><?php the_title(); ?>
To hide the post heading/title , I modified it as follows:
<h1 class="entry-title"><?php if(wp_emember_is_member_logged_in()) the_title(); ?>
The above is a simple example which will suppress the post heading if someone is not logged in.
Get member id and his/her membership level
<?php $emember_auth = Emember_Auth::getInstance(); $user_id = $emember_auth->getUserInfo('member_id'); if (!empty($user_id)) { //User is logged in so add your conditional code here $membership_level = $emember_auth->getUserInfo('membership_level'); if($membership_level == 1) { //Add stuff for this level } } ?>
Get the homepage URL from the logged in member’s membership level
<?php $emember_auth = Emember_Auth::getInstance(); $user_id = $emember_auth->getUserInfo('member_id'); if (!empty($user_id)) { //User is logged in so add your conditional code here $membership_level_resultset = $emember_auth->userInfo->primary_membership_level; $home_page_url = $membership_level_resultset->loginredirect_page; //Do something with this URL } ?>
Show Logged in Member’s Username or other details
<?php $emember_auth = Emember_Auth::getInstance(); $username = $emember_auth->getUserInfo('user_name'); echo "Username of the logged in member: ".$username; ?>
Check if the Logged in Member is Allowed to See a Post
<?php $emember_auth = Emember_Auth::getInstance(); $post_id = "10" if($emember_auth->is_protected_post($post_id)) { //This member is allowed to see this post }
Retrieve Member’s Data
- How to retrieve logged-in member’s data using a shortcode or PHP code
- How to retrieve a particular member’s data using shortcode or PHP
Retrieve a Member Record Using the Username
The following PHP code will retrieve a member’s record for the given username:
<?php $username = "johndoe";//Replace with the actual username $member = emember_get_member_by_username ($username); echo "Member's First Name: " . $member->first_name; //Output the first name echo "Member's Last Name: " . $member->last_name; //Output the last name ?>
Retrieve Total Members Count
The following PHP code will display the total members count:
<?php echo emember_get_total_members(array()); ?>
Member Registration Completion Hook
If you need to execute some custom code after a member completes the registration, use the following action hook:
Show Different Navigation Menu to your Members and Non-members
Show Logged in Member’s Details Using Shortcode
You can use a shrotcode to show any details of the logged in member on a WordPress post or page. Refer to the eMember shortcode documentation for more details.
Placing a Registration Form For a Particular Membership Level to Give Backdoor Entrance
Free Members Must Confirm Their Email Address
Get the Login Page’s URL from the System
$emember_config = Emember_Config::getInstance(); $login_page_url = $emember_config->getValue('login_page_url');
Get the Registration Page’s URL from the System
$emember_config = Emember_Config::getInstance(); $login_page_url = $emember_config->getValue('eMember_registration_page');
Get the Edit Profile Page’s URL from the System
$emember_config = Emember_Config::getInstance(); $login_page_url = $emember_config->getValue('eMember_profile_edit_page');
How to Manage Content Protection in WP eMember
You can manage the protection of specific posts, pages, categories, comments from the “Manage Content Protection” menu of the eMember plugin. You can also set protection of a post or page while editing it in the WordPress editor.
Quick Summary of Content Protection
You perform the following operations in the “Manage Content Protection” menu to protect content in eMember.
- Lock-down the content you want to protect via General Protection. Select the items you want protected while you have “General Protection” selected from the dropdown menu.
- Then select a membership level from the dropdown menu and select items you want accessible to that membership level. Do this step for all the membership levels.
Content Protection Video Tutorial
Content Protection Written Instruction
It is important to understand how the protection mechanism works. There are two steps to protecting and giving access to specific content:
- First you need to protect the content by selecting “General Protection” from the drop down menu and checking the checkboxes next to the posts, pages, categories,comments that you want to protect (don’t forget to hit the ‘Set Protection’ button).
- Now that you have all the important content locked down, you can start to selectively give access to it according to the membership levels. Select a membership level from the drop down menu and check the checkboxes next to the posts, pages, categories, comments that you want the members of this membership level to be able to view (Don’t forget to hit the Grant Access button).
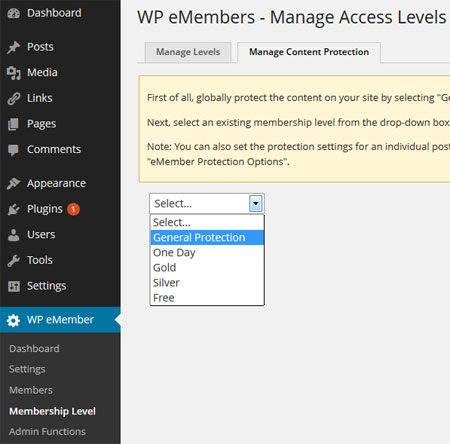
WP eMember Content Protection Management
To protect content for a specific membership level simply select the membership level from the drop down menu and check the checkbox next to the individual posts, pages, categories that you want to give acess to.
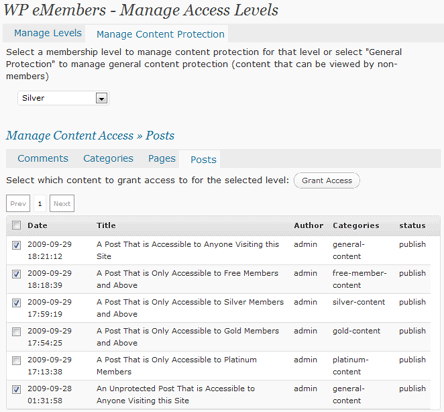
Selective Content Protection for Different Membership Levels
The easiest way to manage content protection is through the protection of “Categories”. It allows you to give access to all the posts or pages under a certain category without having to individually select each post or page. So for example, you can create a category called “Basic” and put all the posts and pages under this category that you want to give access to for the “Basic” Membership level.
Protecting a Section of a Post or Page
Site Wide Content Protection
Protecting Posts or Pages From the Editor
How to Add a Membership Level in WP eMember
WP eMember uses a concept of membership levels. This feature is designed to give you, the site owner, the ability to easily control and assign what content your members can and cannot access on your site.
Table of Contents
- What is a Membership Level
- Creating a Membership Level
- Understanding the Account Expiry Condition
- Managing Remaining Time After Subscription Cancellation (Prorating)
- Video Tutorial
- Additional Resources
What is a Membership Level
When a visitor becomes a member, he is assigned to one of the membership levels you create in the plugin. The member will then have access to the site’s content based on the permissions you defined in the membership level.
The membership level also controls how long a member of that level has access to the content. For example: if you have created a level with a 60 day duration, then members who belong to that level will get access to the content for 60 days from the date they joined. After that time, their account will expire and they will need to renew or upgrade their account.
You can create as many membership levels as you want to. So create a membership level for each different variations of access that you need to offer on your site. Then you can assign members to a membership level based on the kind of access to content and expiry you want for them.
Creating a Membership Level
To add a new membership level, you need to browse to the “Membership Level” menu of the plugin.
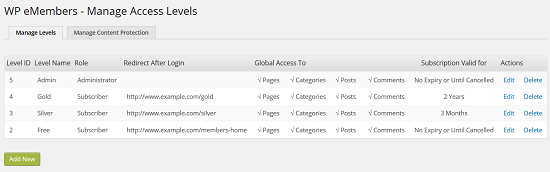
From the “Manage Levels” tab you can click on the “Add New” button to add a new membership level.
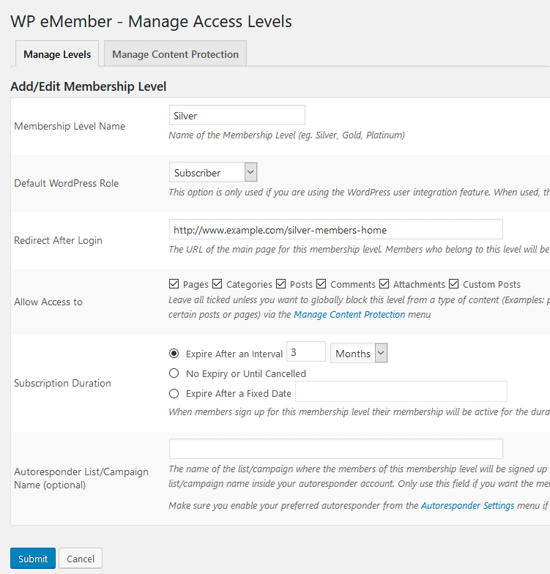
The Membership level addition menu has help text next to each field to guide you. You can edit or delete an existing membership level from this “Manage Levels” interface too.
Understanding the Account Expiry Condition
The expiry of a member’s account is controlled by the membership level assigned to that member. So the value that you set in the Subscription Duration field will control how the account will expire.
Read the following resources to get a better understanding of this so you can set the Subscription Duration value for a membership level according to your needs.
- Manually activating expired account
- Difference between Buy Now and Subscription buttons
- Membership time after subscription cancellation
Managing Remaining Time After Subscription Cancellation (Prorating)
If you are using a subscription-type button for your membership to charge recurring payments and want the member to be able to use their remaining time after the subscription payment agreement is canceled, follow these steps:
To prevent immediate cancellation of a member’s account when a subscription is canceled, avoid using the “No Expiry or Until Cancelled” option for the membership level. Instead, set a duration value that matches or is one day more than the subscription period. This approach ensures that the plugin sets the account to “unsubscribed” upon cancellation, allowing the member to use the remaining subscription duration.
Remaining Time Setup Example:
Let’s say you are creating a subscription payment button to charge every 30 days. You can set a value of 31 days in the subscription duration field of the membership level configuration. The extra day acts as a buffer to ensure the payment gateway can process the recurring charge and notify the plugin before it deactivates the account. This creates a cycle of 31 days. It will ensure that the member will continue to have access for another 31 days after receiving a subscription recurring charge. As long as the recurring charges continue, the member will have access. If the subscription is canceled, the account will expire after the remaining days for the cycle.
Video Tutorial
Read the manage content protection documentation to learn how to apply protection to your content for different membership levels that you create.
Additional Resources
The following documentation is good to read if you want to configure notification email before account expiry: